How to use APIs in a web application?
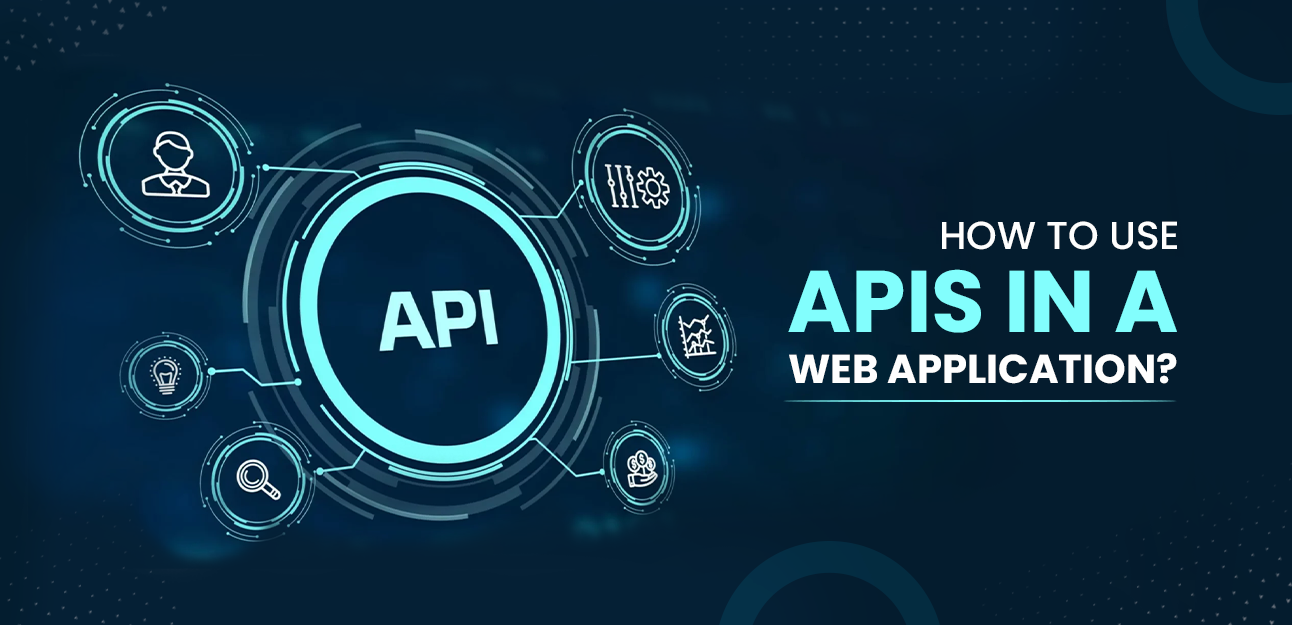
APIs, or Application Programming Interfaces, are essential for modern web applications. They serve as connectors that allow different software systems to communicate with one another, making data exchange, and fetch data from API online.
Key Benefits of Using APIs:
- Enhanced Functionality: By leveraging third-party services, developers can integrate complex features without the need to build them from scratch.
- Improved User Experience: APIs allow for seamless integration of various services, leading to more cohesive and user-friendly applications.
This blog will guide you through a step-by-step approach to integrating API calls into your web applications. We will cover everything from understanding what APIs are and how they work, to practical instructions on sending requests and handling responses. Best practices for integration and common use cases will also be discussed to provide a comprehensive understanding of using APIs effectively.
Analyzing this blog, you will learn what is an API in programming in detail.
Understanding APIs
APIs are essential in modern web development. API full form is an Application Programming Interface. They allow different software systems to communicate and work together smoothly. An API system is like a middleman that enables one application to ask another for data or services, following specific rules and protocols.
How APIs Facilitate Software Communication?
API development act as a connection point between various software systems, offering a standardized method for them to interact. When one application requires certain information or features from another system, it sends an API request. The system receiving the request processes it and either returns the requested data or carries out the specified action.
Different Types of APIs
There are several types of APIs available, each with its unique characteristics and use cases:
- REST (Representational State Transfer): The most commonly used type due to its simplicity and flexibility. RESTful APIs use standard HTTP methods like GET, POST, PUT, and DELETE for communication.
- SOAP (Simple Object Access Protocol): Known for its robustness and security features. It relies on XML messaging for communication.
- GraphQL: Developed by Facebook, this API type allows clients to request exactly the data they need, making it more efficient in terms of data transfer.
- gRPC: A high-performance RPC framework developed by Google. It uses Protocol Buffers for serialization and is ideal for microservices architecture.
Understanding these types helps developers choose the right API connection based on their application's needs and constraints.
By using and calling APIs effectively, developers can enhance their web applications with additional functionalities without reinventing the wheel. This not only speeds up development but also improves user experience by integrating reliable external services seamlessly into their applications.
Key Components Involved in an API Interaction
1. API Client
The API Client represents the user or external application that initiates a request. This could be a web browser, a mobile app, or another server. The client interacts with the API access by sending requests and processing responses.
2. API Key
An API Key is a unique identifier used for authentication and authorization purposes. It ensures that only authorized clients can access the API's services. Typically, this key is included in the request headers or as a query parameter.
3. API Request
The API Request is the message sent from the client to the server. It includes:
- The endpoint URL specifying the resource.
- The HTTP method (e.g., GET, POST) indicating the action to perform.
- Optional parameters and headers providing additional data and context.
4. API Server
The API Server processes incoming requests and generates appropriate responses. It acts as the intermediary between the client and backend resources, handling tasks such as data retrieval, storage, and processing.
5. API Response
The API Response is the data returned by the server to the client. It typically includes:
- A status code indicating success or failure.
- Structured data in formats like JSON or XML.
- Optional headers with additional metadata.
Understanding these components is crucial for effectively integrating APIs into web applications. Each plays a specific role in ensuring smooth communication between software systems.
Step-by-Step Guide to Using APIs in Web Applications
It is important to learn how do website API works. Below is a step-by-step guide on using APIs in web and software applications.
1. Sending Requests to API Endpoints
When it comes to using APIs in web applications, the first step is sending requests to specific endpoints as defined in the API documentation. This process involves making HTTP requests, typically using methods like GET and POST.
Understanding HTTP Methods
- GET Request: Used to retrieve data from a specified resource.
- POST Request: Used to send data to a server to create or update a resource.
These methods are fundamental in interacting with APIs and understanding their use cases can significantly improve your ability to work with various APIs.
Identify the Endpoint URL
Every API has specific endpoints that define where resources are accessible. These endpoints are usually documented in the API's documentation. Here’s an example of how an endpoint might look:
plaintext https://api.example.com/v1/resource
Each part of this URL indicates a specific aspect:
- https://: The protocol used for secure communication.
- api.example.com: The base URL of the API server.
- /v1/resource: The specific path or endpoint within the API.
Making a GET Request
A GET request is straightforward since it primarily involves specifying the endpoint URL. Here’s an example using JavaScript with the Fetch API:
- javascript fetch('https://api.example.com/v1/resource') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
In this example:
- The fetch function sends a GET request to the specified endpoint.
- The response is then converted into JSON format.
- The resulting data is logged to the console or handled as needed.
Making a POST Request
A POST request involves sending additional data in the body of the request. This method is useful when you need to create or update resources on the server. Here’s how you can make a POST request:
javascript fetch('https://api.example.com/v1/resource', { method: 'POST', headers: { 'Content-Type': 'application/json', 'Authorization': 'Bearer YOUR_API_KEY' }, body: JSON.stringify({ key1: 'value1', key2: 'value2' }) }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
In this example:
- The method property specifies that it's a POST request.
- Headers like Content-Type and Authorization provide necessary metadata.
- The body contains data in JSON format, which is sent along with the request.
Importance of API Documentation
API documentation is crucial as it provides comprehensive details about available endpoints, required parameters, expected responses, and any authentication mechanisms. Always refer to the API documentation before making requests to ensure correct usage and adherence to guidelines.
By mastering these fundamental steps—understanding HTTP methods, identifying endpoint URLs, making GET and POST requests—you lay a solid foundation for effectively integrating APIs into your web applications.
2. Handling Responses from APIs
When an API server processes an HTTP request, it sends back a response that includes both a status code and data. The data is typically formatted in either JSON (JavaScript Object Notation) or XML (eXtensible Markup Language).
Common Data Formats
- JSON: A lightweight data-interchange format that is easy for humans to read and write, and easy for machines to parse and generate. Most modern web applications prefer JSON due to its simplicity and efficiency.
- XML: A markup language that defines rules for encoding documents in a format that is both human-readable and machine-readable. While more verbose than JSON, XML remains popular in legacy systems and certain industries.
Handling JSON Responses
JSON responses are straightforward to handle in most programming languages. For instance, in JavaScript, you can use the fetch API to send a GET request to an endpoint URL and then process the returned JSON data:
javascript fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { console.log(data); }) .catch(error => { console.error('Error:', error); });
Handling XML Responses
Processing XML responses requires parsing the XML structure. In JavaScript, this can be done using the DOMParser object:
- javascript fetch('https://api.example.com/data') .then(response => response.text()) .then(xmlString => { const parser = new DOMParser(); const xmlDoc = parser.parseFromString(xmlString, 'application/xml'); console.log(xmlDoc); }) .catch(error => { console.error('Error:', error); });
Understanding how to handle these common data formats is crucial for integrating APIs into your web application effectively. It allows you to manipulate and display the data as needed, enhancing the functionality and user experience of your application.
By mastering the handling of API responses, developers unlock the full potential of integrating diverse services into their web applications.
3. Testing APIs Before Integration
Testing APIs before integrating them into your web application is crucial to ensure they work as expected and meet your requirements. Tools like Postman are invaluable for this purpose.
Postman offers a user-friendly interface for crafting and sending HTTP requests, such as GET and POST, to API endpoints. By entering the endpoint URL and selecting the desired HTTP method, you can simulate requests and observe the responses returned by the API server.
Key features of Postman include:
- Request Building: Easily configure request headers, parameters, and body content.
- Environment Variables: Manage multiple environments (e.g., development, production) with different configurations.
- Collections: Organize API requests into collections for better manageability.
- Automated Testing: Write tests using JavaScript to automatically validate responses.
Other useful tools for API testing:
- Insomnia: Another powerful tool with an intuitive interface for testing RESTful APIs.
- Swagger UI: Provides a visual interface for interacting with APIs defined by OpenAPI specifications.
By leveraging these tools, you can verify that your API interactions are functioning correctly before moving on to full integration in your web application. This step helps catch potential issues early, ensuring smoother development and deployment processes.
Conclusion
Ensuring secure interactions with APIs is crucial for safeguarding data and maintaining trust. A unique identifier provided to the client, which must be included in requests to authenticate and authorize actions. Often used in OAuth protocols, tokens provide a more secure way of managing sessions and permissions.
Using these authentication mechanisms helps verify the identity of the client and ensures that only authorized users can access specific endpoints. Implementing these security measures not only protects your web application but also enhances user trust by ensuring their data is handled securely.